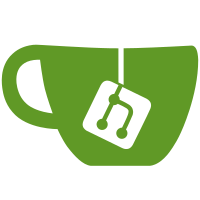
This will let us implement common functions seen in other lisps, and allow them to be importable, without explicit imports. The goal is to keep this as small as we can; we don't want too much magic. I've added `take' and `drop' as examples of what we can do.
23 lines
545 B
Hy
23 lines
545 B
Hy
;;;; This contains some of the core Hy functions used
|
|
;;;; to make functional programming slightly easier.
|
|
;;;;
|
|
|
|
|
|
(defn take [count what]
|
|
"Take `count` elements from `what`, or the whole set if the total
|
|
number of entries in `what` is less than `count`."
|
|
(setv what (iter what))
|
|
(for [i (range count)]
|
|
(yield (next what))))
|
|
|
|
|
|
(defn drop [count coll]
|
|
"Drop `count` elements from `coll` and return the iter"
|
|
(let [ [citer (iter coll)] ]
|
|
(for [i (range count)]
|
|
(next citer))
|
|
citer))
|
|
|
|
|
|
(def *exports* ["take" "drop"])
|